React useFetch hook
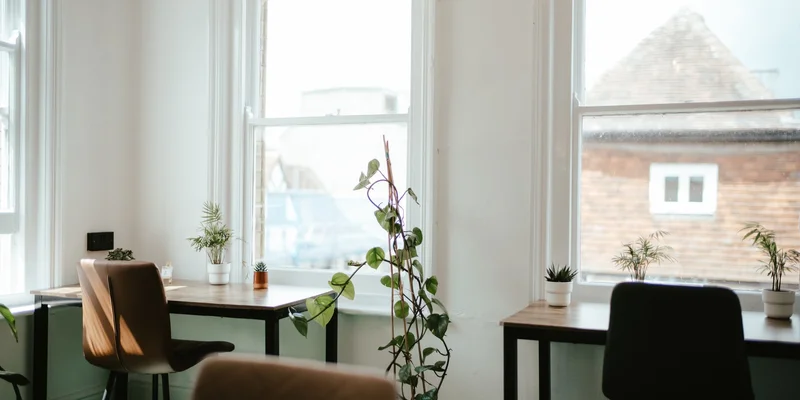
Implements fetch()
in a declarative manner.
- Create a custom hook that takes a
url
andoptions
. - Use the
useState()
hook to initialize theresponse
,error
andabort
state variables. - Use the
useEffect()
hook to asynchronously callfetch()
and update the state variables accordingly. - Create and use an
AbortController
to allow aborting the request. Use it to cancel the request when the component unmounts. - Return an object containing the
response
,error
andabort
state variables.
const useFetch = (url, options) => { const [response, setResponse] = React.useState(null); const [error, setError] = React.useState(null); const [abort, setAbort] = React.useState(() => {}); React.useEffect(() => { const fetchData = async () => { try { const abortController = new AbortController(); const signal = abortController.signal; setAbort(abortController.abort); const res = await fetch(url, {...options, signal}); const json = await res.json(); setResponse(json); } catch (error) { setError(error); } }; fetchData(); return () => { abort(); } }, []); return { response, error, abort }; }; const ImageFetch = props => { const res = useFetch('https://dog.ceo/api/breeds/image/random', {}); if (!res.response) { return <div>Loading...</div>; } const imageUrl = res.response.message; return ( <div> <img src={imageUrl} alt="avatar" width={400} height="auto" /> </div> ); }; ReactDOM.createRoot(document.getElementById('root')).render( <ImageFetch /> );