Programmatically trigger event on HTML element using JavaScript
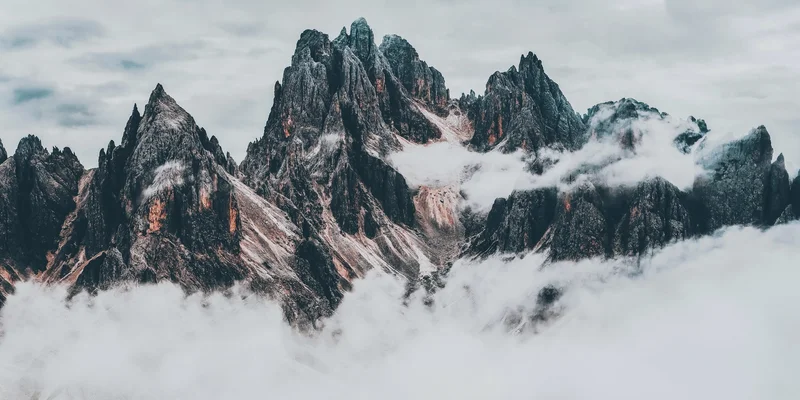
JavaScript's EventTarget.dispatchEvent()
method allows you to trigger an event programmatically. This method accepts an Event
object as its only argument, which can be created using either the regular Event
constructor or the CustomEvent
constructor.
Usually, you'd want to use the CustomEvent
constructor, as it allows you to pass custom data to the event listener. The constructor accepts two arguments: eventType
and detail
. The eventType
argument is a string that specifies the type of event to be created (e.g. 'click'
). The detail
argument is an object that contains the custom data you want to pass to the event listener.
Putting it all together, you can create a function that programmatically triggers an event on an HTML element:
const triggerEvent = (el, eventType, detail) =>
el.dispatchEvent(new CustomEvent(eventType, { detail }));
const myElement = document.getElementById('my-element');
myElement.addEventListener('click', e => console.log(e.detail));
triggerEvent(myElement, 'click');
// The event listener will log: null
triggerEvent(myElement, 'click', { username: 'bob' });
// The event listener will log: { username: 'bob' }