React useClickInside hook
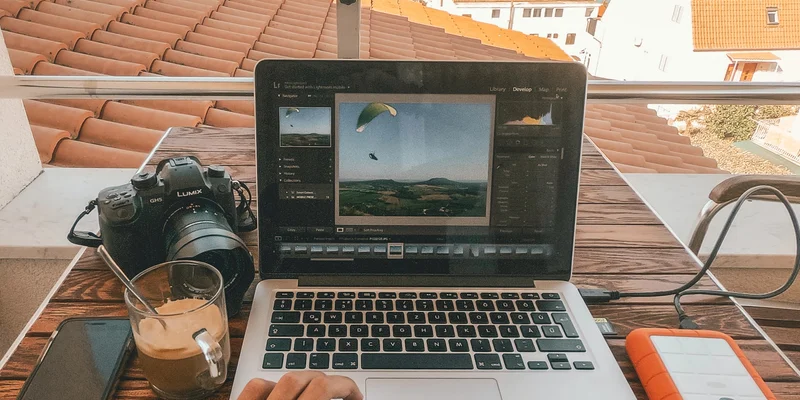
Handles the event of clicking inside the wrapped component.
- Create a custom hook that takes a
ref
and acallback
to handle the'click'
event. - Use the
useEffect()
hook to append and clean up theclick
event. - Use the
useRef()
hook to create aref
for your click component and pass it to theuseClickInside
hook.
const useClickInside = (ref, callback) => { const handleClick = e => { if (ref.current && ref.current.contains(e.target)) { callback(); } }; React.useEffect(() => { document.addEventListener('click', handleClick); return () => { document.removeEventListener('click', handleClick); }; }); }; const ClickBox = ({ onClickInside }) => { const clickRef = React.useRef(); useClickInside(clickRef, onClickInside); return ( <div className="click-box" ref={clickRef} style={{ border: '2px dashed orangered', height: 200, width: 400, display: 'flex', justifyContent: 'center', alignItems: 'center' }} > <p>Click inside this element</p> </div> ); }; ReactDOM.createRoot(document.getElementById('root')).render( <ClickBox onClickInside={() => alert('click inside')} /> );