React useClickOutside hook
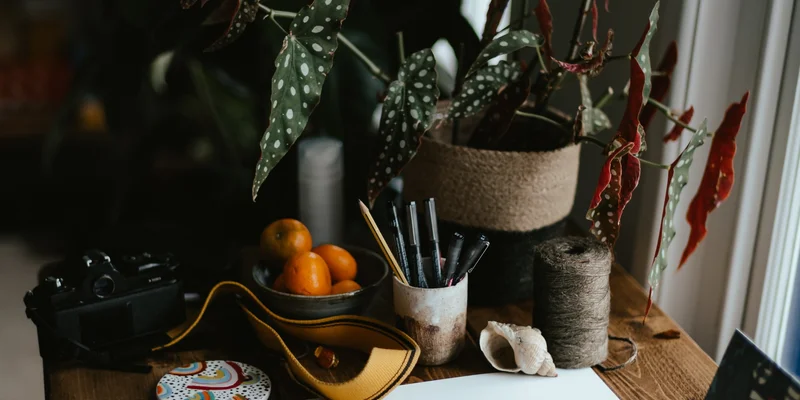
Handles the event of clicking outside of the wrapped component.
- Create a custom hook that takes a
ref
and acallback
to handle theclick
event. - Use the
useEffect()
hook to append and clean up theclick
event. - Use the
useRef()
hook to create aref
for your click component and pass it to theuseClickOutside
hook.
const useClickOutside = (ref, callback) => { const handleClick = e => { if (ref.current && !ref.current.contains(e.target)) { callback(); } }; React.useEffect(() => { document.addEventListener('click', handleClick); return () => { document.removeEventListener('click', handleClick); }; }); }; const ClickBox = ({ onClickOutside }) => { const clickRef = React.useRef(); useClickOutside(clickRef, onClickOutside); return ( <div className="click-box" ref={clickRef} style={{ border: '2px dashed orangered', height: 200, width: 400, display: 'flex', justifyContent: 'center', alignItems: 'center' }} > <p>Click out of this element</p> </div> ); }; ReactDOM.createRoot(document.getElementById('root')).render( <ClickBox onClickOutside={() => alert('click outside')} /> );