React useCopyToClipboard hook
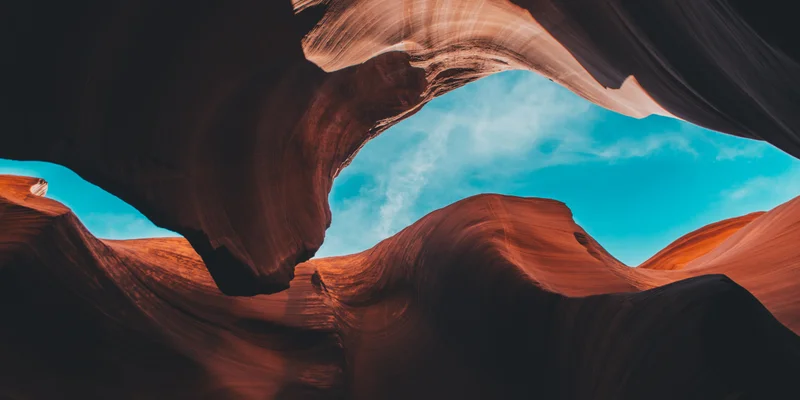
Copies the given text to the clipboard.
- Use the copyToClipboard snippet to copy the text to clipboard.
- Use the
useState()
hook to initialize thecopied
variable. - Use the
useCallback()
hook to create a callback for thecopyToClipboard
method. - Use the
useEffect()
hook to reset thecopied
state variable if thetext
changes. - Return the
copied
state variable and thecopy
callback.
const useCopyToClipboard = text => { const copyToClipboard = str => { const el = document.createElement('textarea'); el.value = str; el.setAttribute('readonly', ''); el.style.position = 'absolute'; el.style.left = '-9999px'; document.body.appendChild(el); const selected = document.getSelection().rangeCount > 0 ? document.getSelection().getRangeAt(0) : false; el.select(); const success = document.execCommand('copy'); document.body.removeChild(el); if (selected) { document.getSelection().removeAllRanges(); document.getSelection().addRange(selected); } return success; }; const [copied, setCopied] = React.useState(false); const copy = React.useCallback(() => { if (!copied) setCopied(copyToClipboard(text)); }, [text]); React.useEffect(() => () => setCopied(false), [text]); return [copied, copy]; }; const TextCopy = props => { const [copied, copy] = useCopyToClipboard('Lorem ipsum'); return ( <div> <button onClick={copy}>Click to copy</button> <span>{copied && 'Copied!'}</span> </div> ); }; ReactDOM.createRoot(document.getElementById('root')).render( <TextCopy /> );