React useSet hook
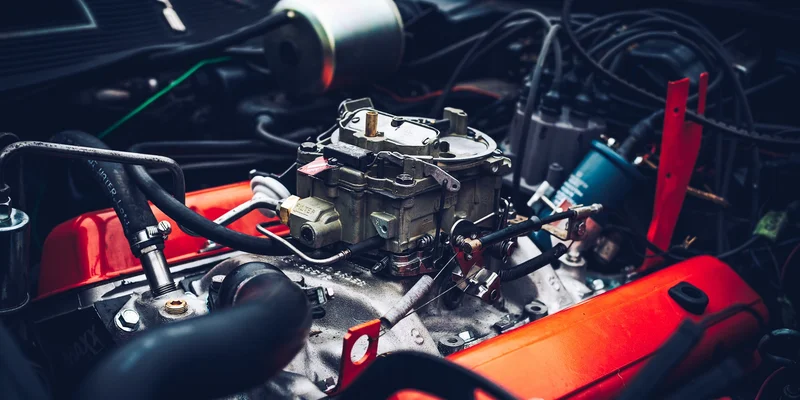
Creates a stateful Set
object, and a set of functions to manipulate it.
- Use the
useState()
hook and theSet
constructor to create a newSet
from theinitialValue
. - Use the
useMemo()
hook to create a set of non-mutating actions that manipulate theset
state variable, using the state setter to create a newSet
every time. - Return the
set
state variable and the createdactions
.
const useSet = initialValue => { const [set, setSet] = React.useState(new Set(initialValue)); const actions = React.useMemo( () => ({ add: item => setSet(prevSet => new Set([...prevSet, item])), remove: item => setSet(prevSet => new Set([...prevSet].filter(i => i !== item))), clear: () => setSet(new Set()), }), [setSet] ); return [set, actions]; }; const MyApp = () => { const [set, { add, remove, clear }] = useSet(new Set(['apples'])); return ( <div> <button onClick={() => add(String(Date.now()))}>Add</button> <button onClick={() => clear()}>Reset</button> <button onClick={() => remove('apples')} disabled={!set.has('apples')}> Remove apples </button> <pre>{JSON.stringify([...set], null, 2)}</pre> </div> ); }; ReactDOM.createRoot(document.getElementById('root')).render( <MyApp /> );